Collections
Guides
Project Logs
Times
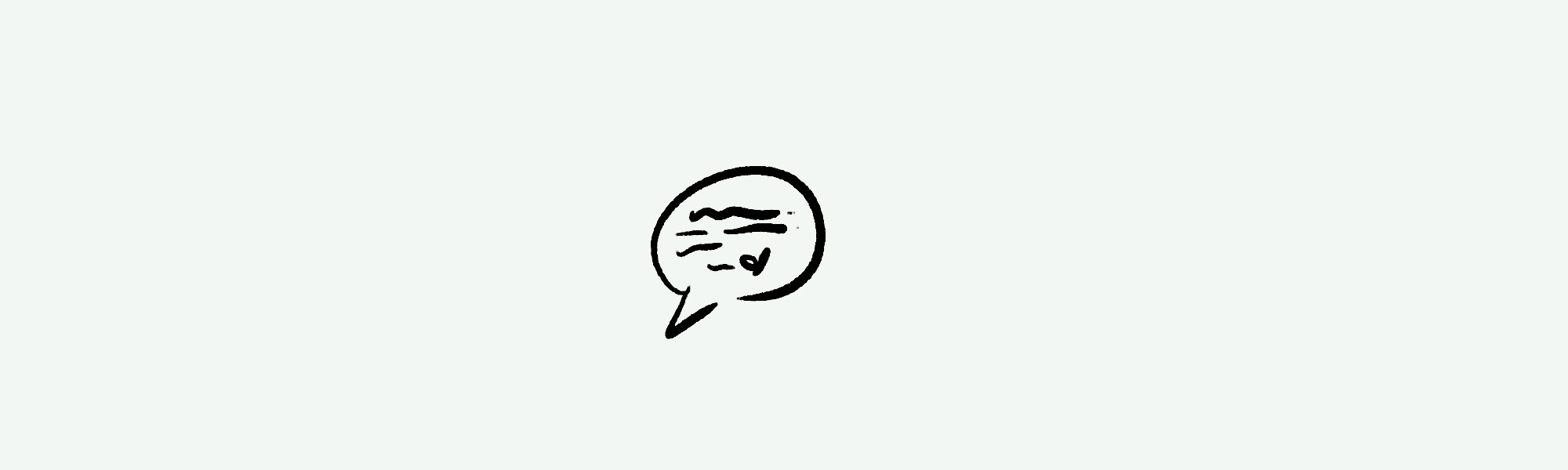
Ongoing
Rust Log
Learning through advent of code (probably a bad idea)
General
Libraries
File
- Struct defined in std::fs
Keywords
mut
- Mutable, variable can be changed after initially set. Variables are immutable by default. Indicating mutability helps prevent bugs at compile time
&mut
- Create mutable reference to a value, whether or not variable can be reassigned.
Types
Option<T>
- All types are either Some(T) (an element of type T exists) or N (No element of type T exists)
Result<T,E>
- Similar to option, but takes in result of computation. Can output either Ok(T) (success) or Err(E) (fail)
Methods
.unwrap()
- Can be called on Option or Result type. If Option
is None
or Result
is Err(E)
, then the program will panic
Traits
#![feature(never_type)]
- Indicating that the function will never return
Other Weird things
- ! delineates macros and not functions
- Adding
b
at the beginning of aprintln
orstdout
, makes your output a byte string instead of a Unicode scalar value. Most of the time this doesn't super matter as long as you are consistent, butu8
values can be preferred because they are always 8 bits, vs Unicode scalar values can be up to 32 bits usize
used for any indices- If you don't use
&
when referencing a value, you are taking ownership of it
Embedded Systems
General Information
-
#![no_std]
and#![no_main]
aren't available, uselibcore
instead- Still have access to primitive types (u8, i32, f64), string slices, time, arrays, and iterators
- Not pulling in other libraries at least means compile times are fast
-
You usually don't have memory allocation, your code is the only thing running
-
No threads, no networking, no
std::fs
-
Using generic HALs to apply functionality to known characteristics like
Input
andOutput
, and then using chip specific libraries to plug into generic HAL for interoperability -
Within USBs, there are different classes. Eg for keyboards it is the HID (Human Interface Device) class